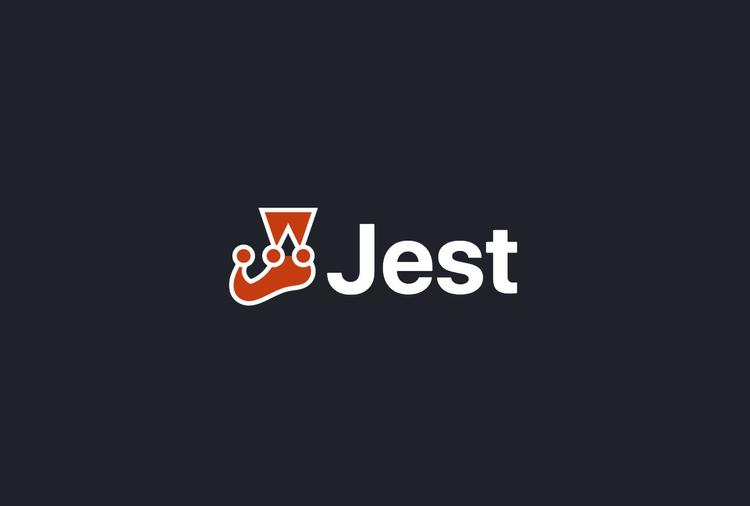
Using @testing-library/react-native in react-native
Learn how to use @testing-library/react-native with a simple unit test. Our insights and experiences.
First, lets get the necessary packages installed:
npm i @testing-library/react-native
or
yarn add @testing-library/react-native
This library can be viewed on npmjs.com at
@testing-library/react-native
Here's a simple example of unit testing a React Native component using jest
and react-native-testing-library
:
import React from 'react';
import { render, fireEvent } from '@testing-library/react-native';
import MyComponent from './MyComponent';
describe('MyComponent', () => {
it('renders correctly', () => {
const { getByText } = render(<MyComponent />);
expect(getByText('Hello World')).toBeTruthy();
});
it('handles button press', () => {
const { getByText } = render(<MyComponent />);
const button = getByText('Press Me');
fireEvent.press(button);
expect(getByText('Button Pressed')).toBeTruthy();
});
});
In this example, MyComponent
is being tested for correct rendering and button press handling. The render
function from react-native-testing-library
is used to render the component, and fireEvent.press
is used to simulate a press event on the button. The getByText
method is used to query elements in the component by their text content. The expect
function is used to make assertions about the component's state.